Understanding JavaScript Closures
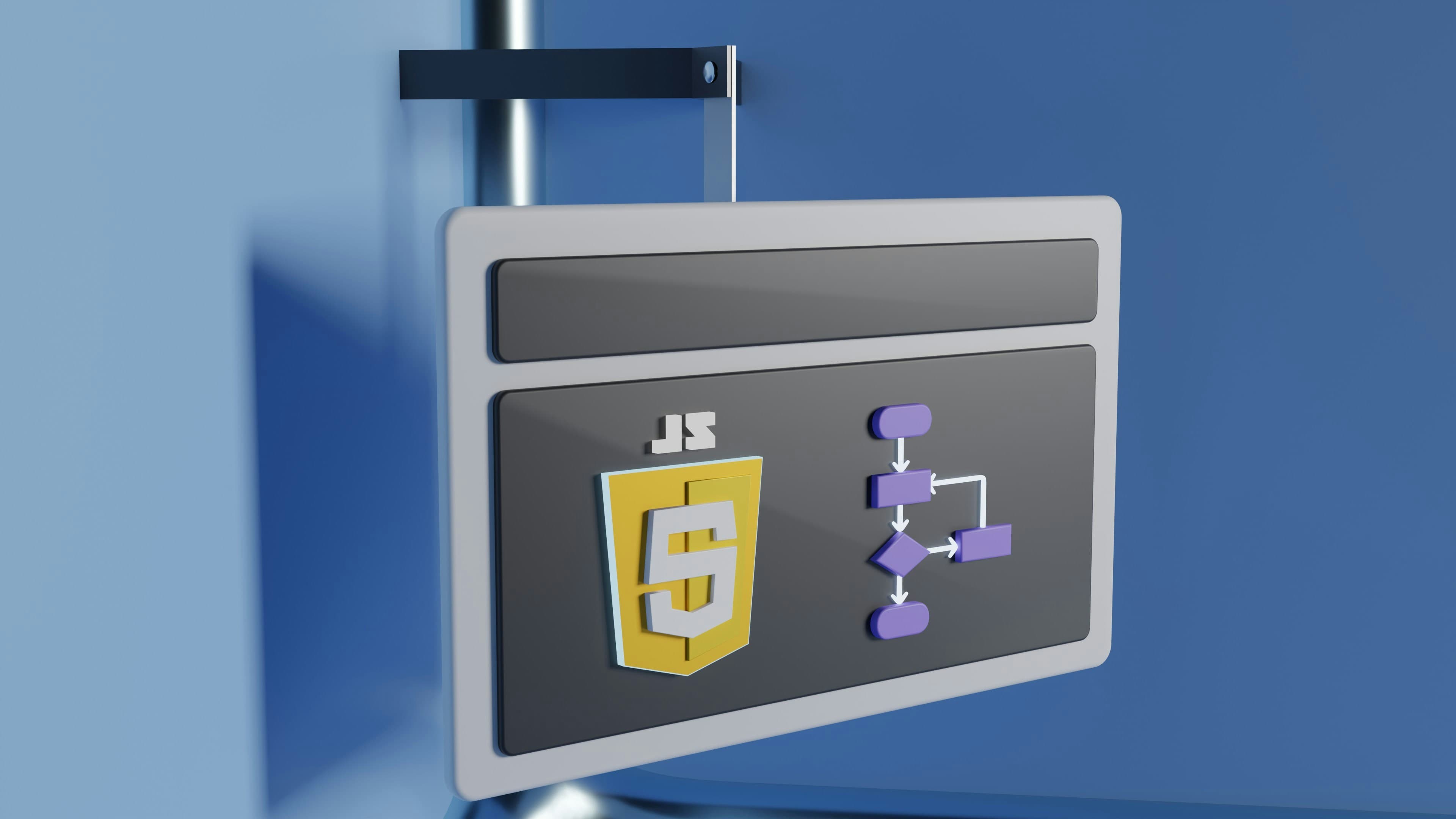
Written by Massa Medi| January 6, 2025
Closures are one of the most powerful yet often misunderstood features of JavaScript. They allow developers to write efficient, modular, and reusable code. But if you're new to programming, they can be tricky to grasp at first. In this blog, we'll explore closures in depth—what they are, how they work, and where you can use them in your JavaScript applications. By the end, you'll have a solid understanding of closures and how to leverage them to improve your code's efficiency and readability.
What Are JavaScript Closures?
At its core, a closure is a function that has access to variables from another function’s scope. In other words, a closure is created when a function "remembers" the environment in which it was created, even after that function has finished executing. Closures allow functions to maintain access to variables from their outer scope.
Here's a simple example of a closure:
function outerFunction() {
let outerVariable = "I'm outside!";
function innerFunction() {
console.log(outerVariable);
}
return innerFunction;
}
const closure = outerFunction();
closure(); // Output: I'm outside!
In this example, the innerFunction
can access theouterVariable
, even though outerFunction
has already finished executing. This is because the inner function forms a closure around the outer function’s variables, keeping them in memory.
How Do Closures Work?
Closures work by creating a reference to the outer function’s variables, even after the outer function has returned. When JavaScript sees that a function is being returned or passed around, it keeps a reference to the variables in the surrounding lexical scope, ensuring that they are available whenever the inner function is invoked.
In technical terms, a closure is created when an inner function accesses a variable that is defined in its outer scope. This "remembering" of variables is what makes closures so powerful.
Practical Use Cases for Closures
Closures aren’t just a theoretical concept—they have many practical applications in real-world JavaScript development. Here are a few examples:
1. Data Encapsulation
Closures are commonly used to encapsulate data and create private variables. This is particularly useful in scenarios where you want to protect certain data from being accessed or modified directly.
function createCounter() {
let count = 0;
return function() {
count++;
console.log(count);
}
}
const counter = createCounter();
counter(); // Output: 1
counter(); // Output: 2
In this example, the count
variable is private to the closure, and only the returned function can access or modify it. This ensures that the variable is not tampered with outside of the closure.
2. Maintaining State in Asynchronous Functions
Closures are also valuable when working with asynchronous code, such as callbacks, timers, or event listeners. They allow you to "remember" values even after an asynchronous operation has completed.
for (let i = 1; i <= 3; i++) {
setTimeout(function() {
console.log(i);
}, 1000 * i);
}
// Output: 1 2 3 (each printed after 1 second, 2 seconds, and 3 seconds)
Thanks to closures, each function retains the correct value of i
, even though the loop has finished running before thesetTimeout
callbacks are executed.
3. Function Factories
Closures can be used to generate functions dynamically based on certain conditions. This is commonly referred to as "function factories."
function createMultiplier(multiplier) {
return function(number) {
return number * multiplier;
}
}
const double = createMultiplier(2);
const triple = createMultiplier(3);
console.log(double(5)); // Output: 10
console.log(triple(5)); // Output: 15
In this case, each function created by createMultiplier
remembers the value of multiplier
, allowing you to create specialized functions like double
and triple
that retain specific behaviors.
Common Pitfalls When Using Closures
While closures are a powerful tool, they can also lead to issues if not used correctly. Here are some common pitfalls to be aware of:
1. Memory Leaks
Closures can unintentionally cause memory leaks by keeping references to variables that are no longer needed. If a closure holds on to variables that are not being used, it can prevent the JavaScript engine from cleaning up memory, leading to performance issues.
2. Debugging Complexity
Debugging closures can be tricky, especially in large applications. Because closures retain access to variables from their outer scope, it can be challenging to track down where specific values are being modified.
Conclusion
Closures are an essential concept in JavaScript that allow you to write more efficient, modular, and reusable code. Whether you're managing private variables, handling asynchronous tasks, or creating function factories, closures give you the power to maintain state and manage scope effectively. While they can be tricky to understand at first, mastering closures will greatly enhance your JavaScript programming skills.
Recommended
Explore More Recent Blog Posts
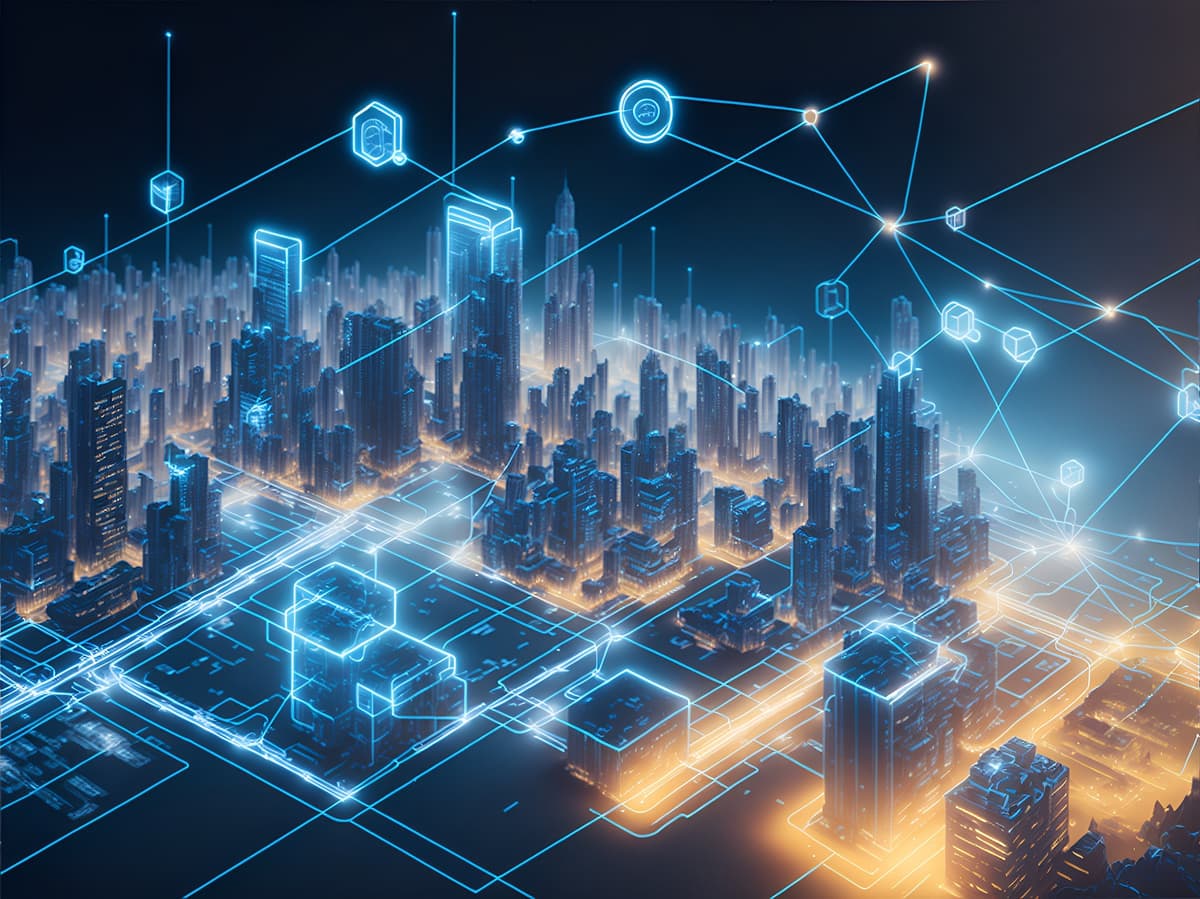
Understanding How the Internet Works
January 13, 2025
Learn how the internet functions, from the basics of networking to protocols like HTTP. Understand key concepts like IP addresses, DNS, and data packet routing.
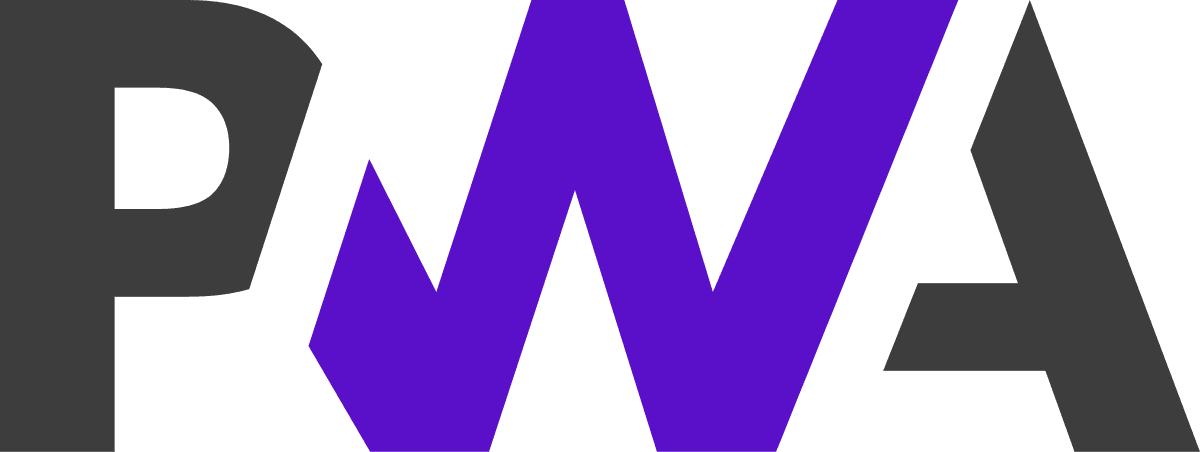
Progressive Web Apps: A Step-by-Step Guide
January 14, 2025
Learn the essentials of building Progressive Web Apps (PWAs) that combine the best of web and mobile apps. This guide covers service workers, manifest files, and offline capabilities to create a seamless user experience.
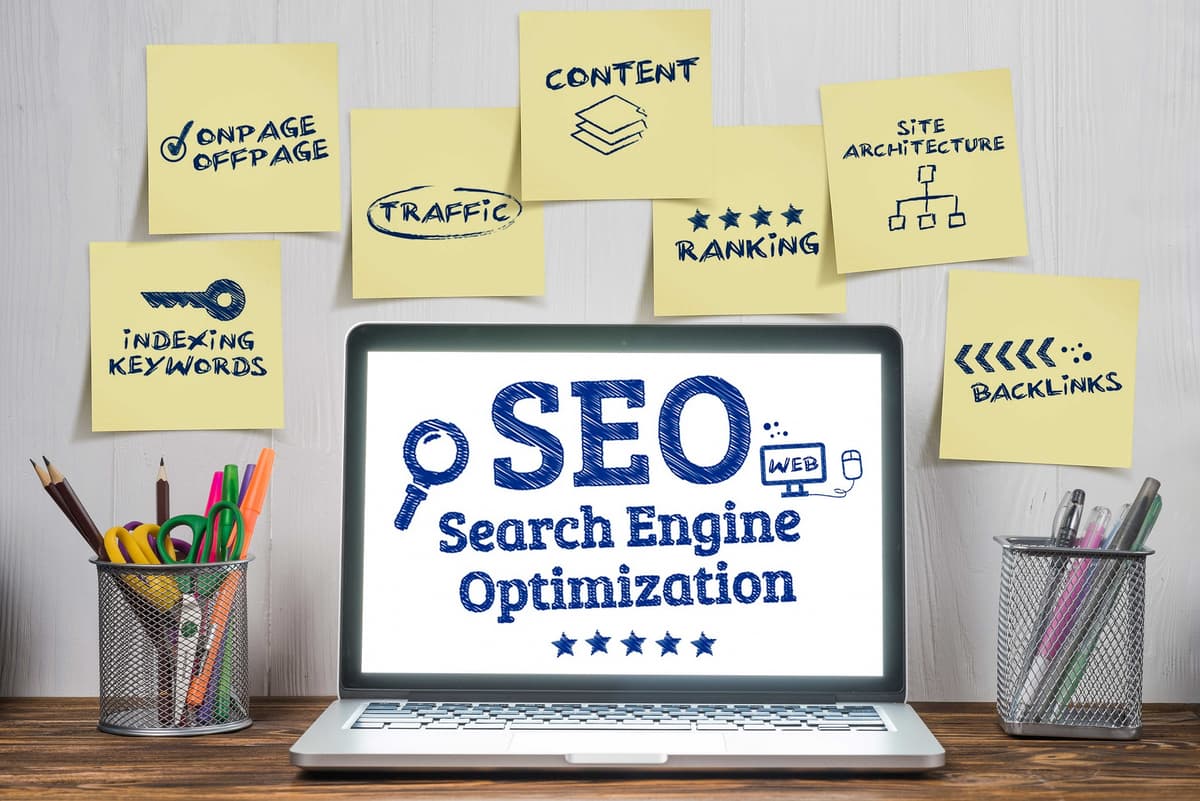
Mastering SEO and Content Marketing Strategies
January 10, 2025
Unlock the secrets of search engine optimization (SEO) and content marketing. Understand how Google indexes pages and learn to create valuable content that attracts and engages your target audience.
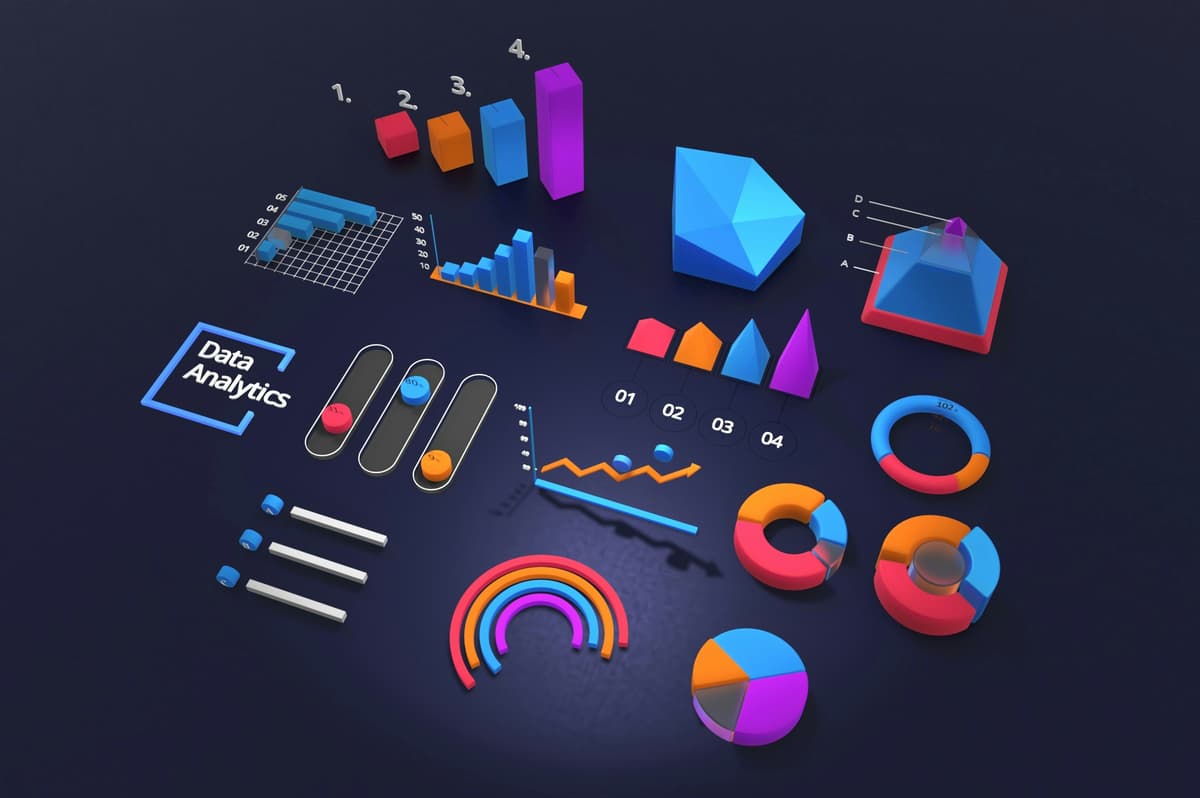
Web Analytics: Tracking User Behavior for Better UX
January 8, 2025
Harness the power of web analytics to improve your website's user experience. Learn how to set up Google Analytics, interpret user behavior data, and use tools like Hotjar and Crazy Egg to optimize your site's performance and conversion rates.
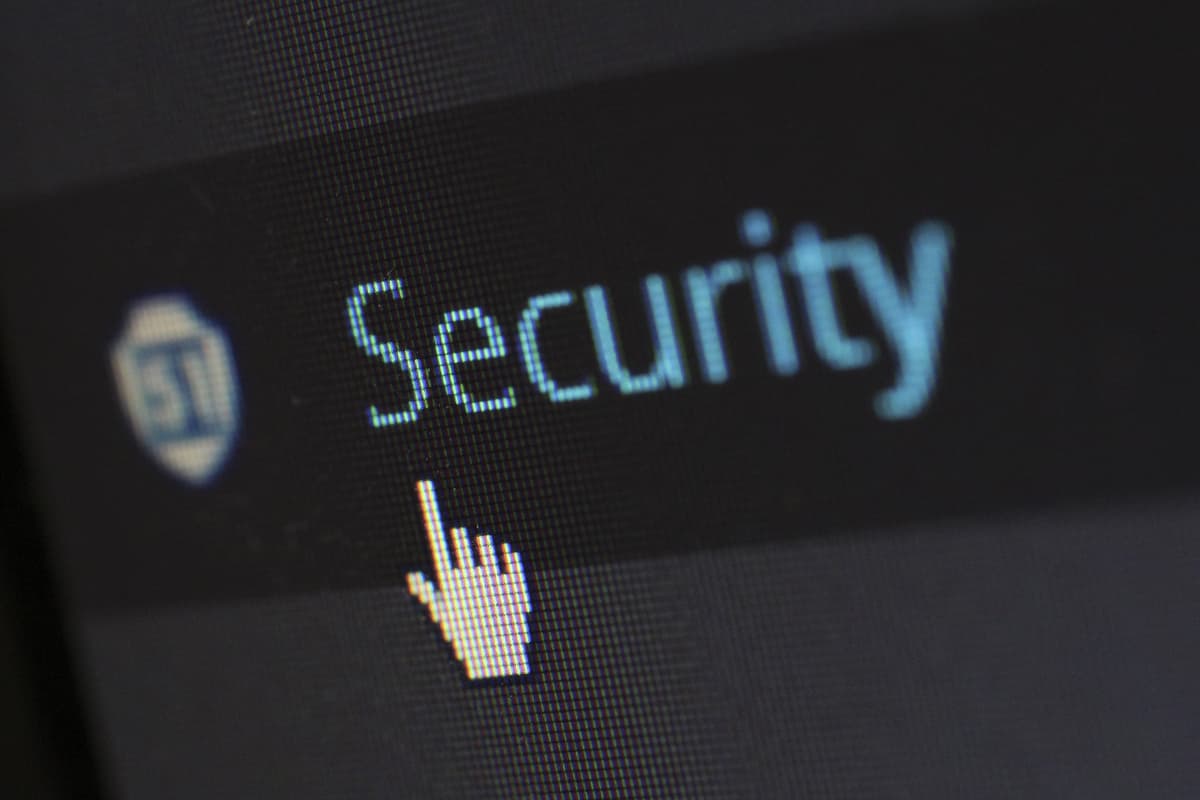
Cybersecurity Essentials for Web Developers
January 4, 2025
Protect your website and users with essential cybersecurity practices. Explore the importance of HTTPS, SSL certificates, and learn about common vulnerabilities like XSS and CSRF.
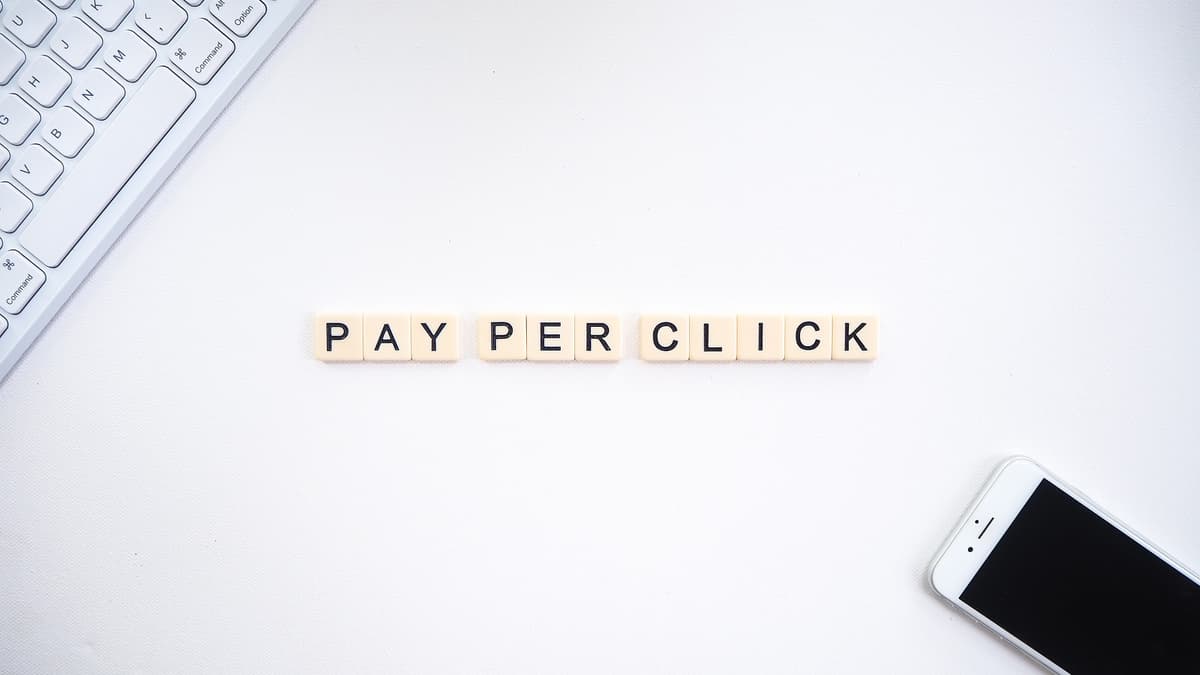
Monetization Strategies for Tech Professionals
January 1, 2025
Explore various ways to monetize your tech skills. From freelancing on platforms like Upwork and Fiverr to creating and selling digital products.
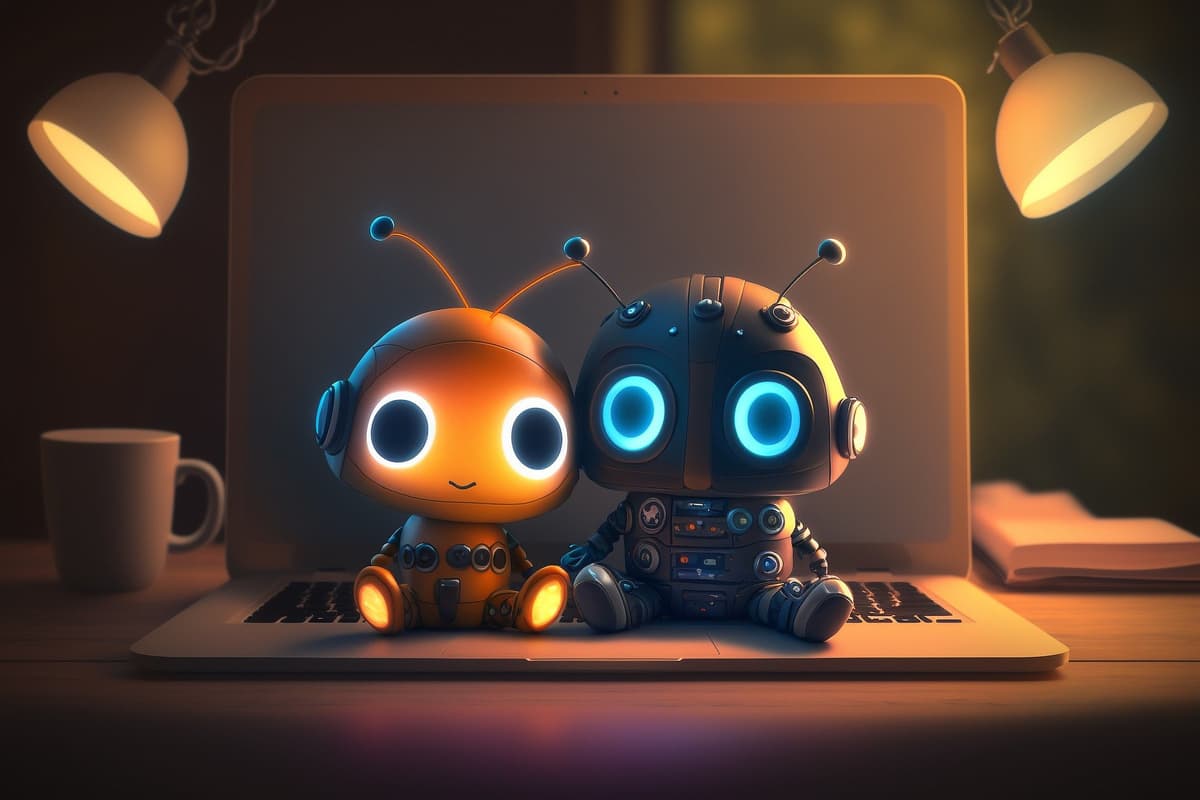
AI Tools for Developers: Boosting Productivity and Creativity
January 12, 2025
Leverage the power of AI to enhance your development workflow. Discover how tools like ChatGPT and GitHub Copilot can assist in coding, content creation, and problem-solving.
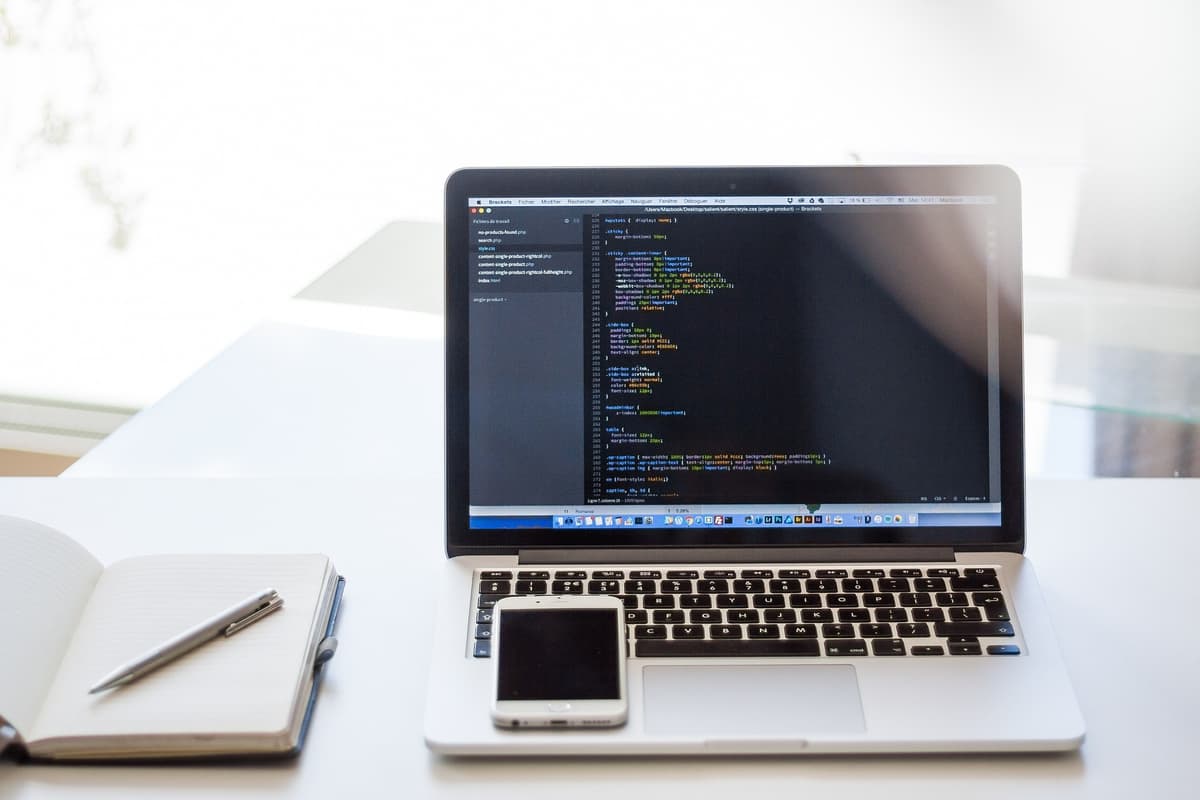
The Rise of No-Code and Low-Code Platforms
January 14, 2025
Explore the growing trend of no-code and low-code platforms. Understand how these tools are changing the landscape of web development and enabling non-technical users to create sophisticated applications.
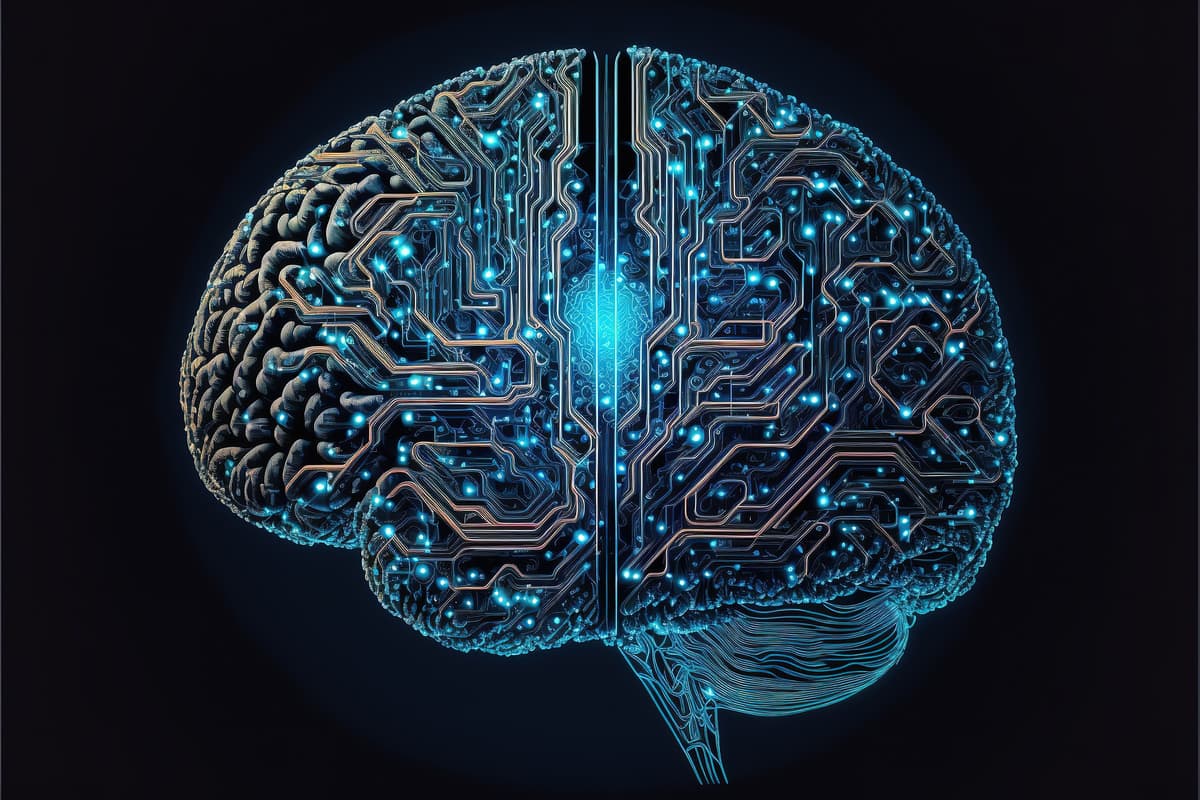
Blockchain and Web3: The Future of the Internet
January 3, 2025
Dive into the world of blockchain technology and Web3. Understand the fundamentals of decentralized applications (dApps), smart contracts, and cryptocurrencies.
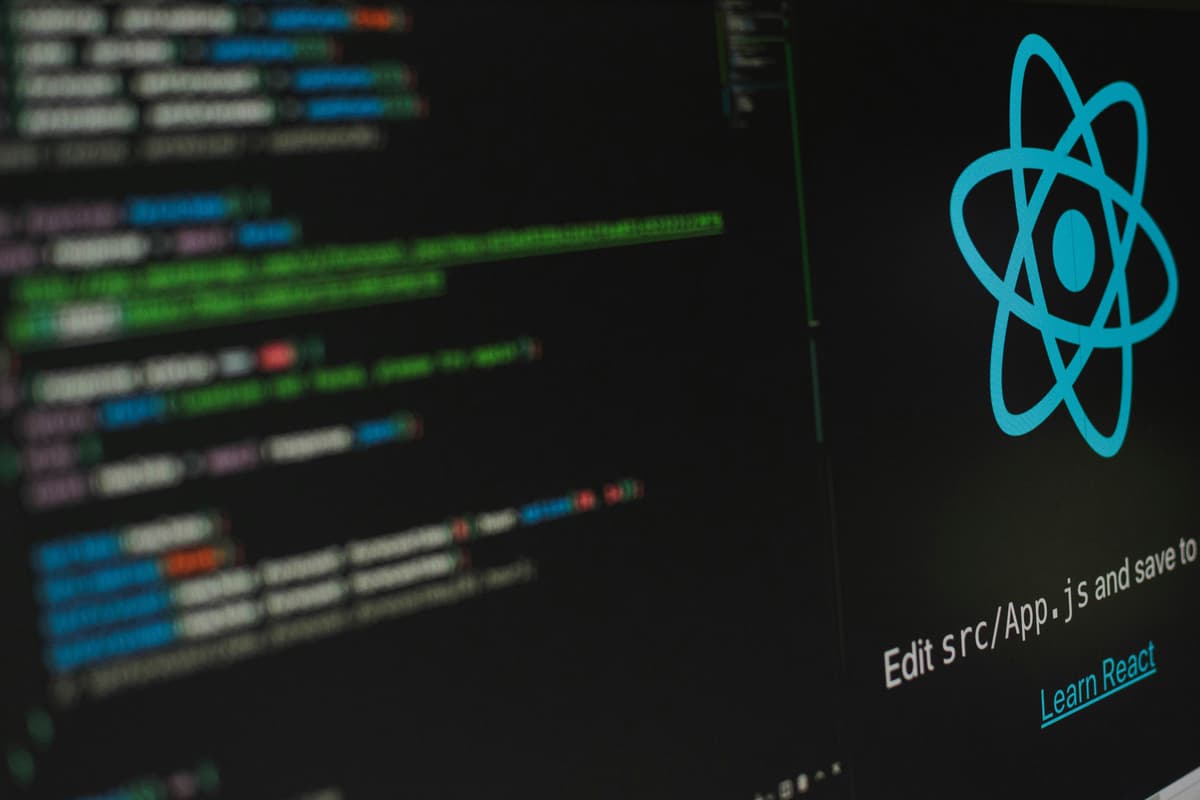
Why Learn React in 2025?
January 13, 2025
Explore the reasons behind React's enduring popularity in 2024. Learn about its efficiency, component-based architecture, and the vibrant ecosystem of libraries that support modern web development.
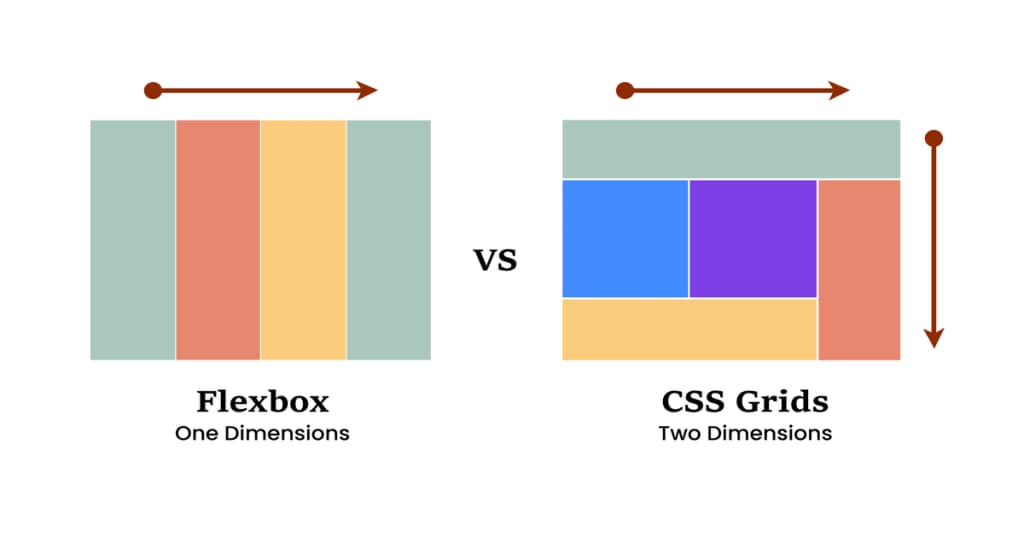
CSS Grid vs. Flexbox: Which to Choose?
January 2, 2025
Understand the differences between CSS Grid and Flexbox, two powerful layout systems in modern web design. This post will help you decide which tool to use based on your project's needs.
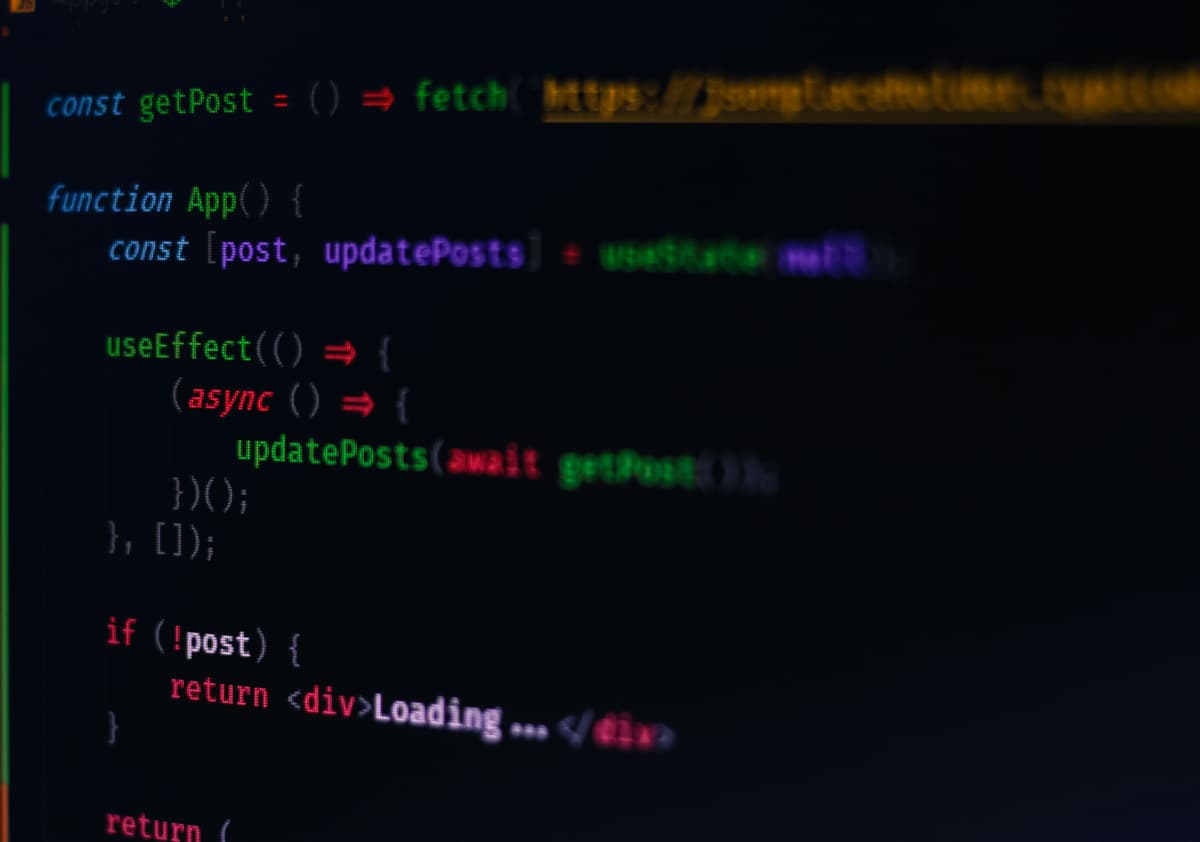
React Hooks: A Comprehensive Guide
January 7, 2025
Get a thorough understanding of React Hooks and how they revolutionize state management in functional components. Explore hooks like useState, useEffect, and custom hooks.
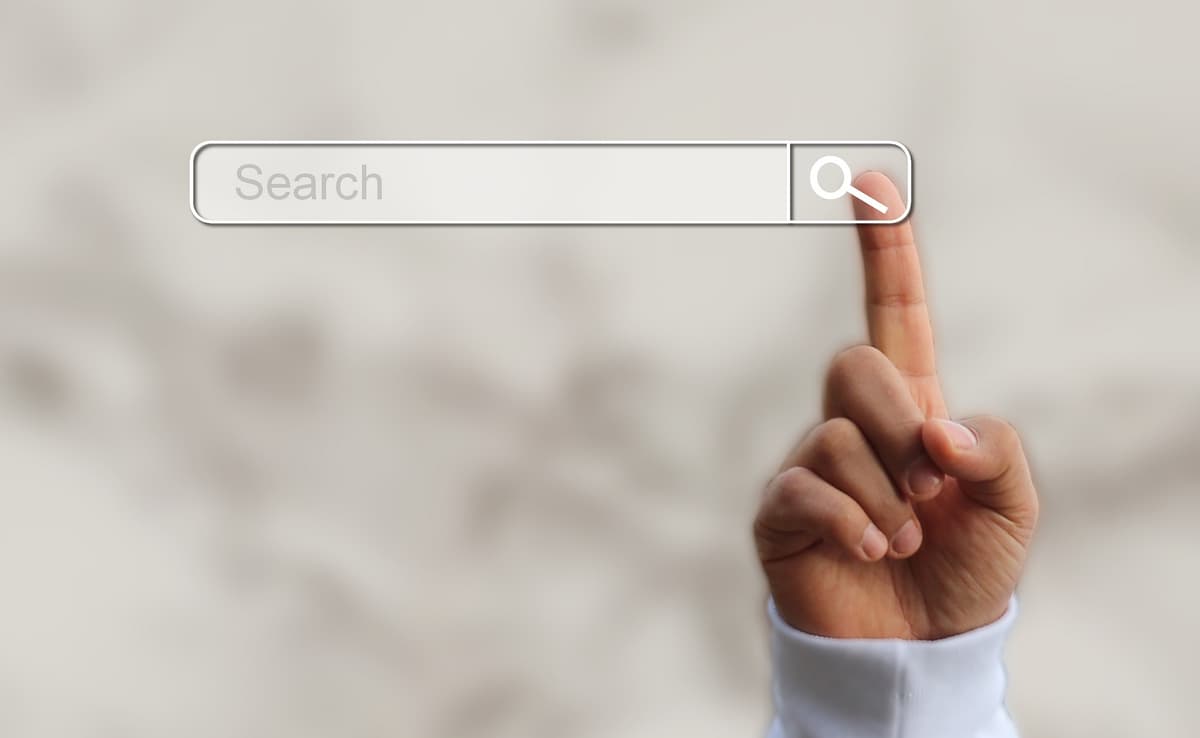
The Ultimate Guide to Google Search Console in 2024
January 7, 2025
Navigate the features and functionalities of Google Search Console to enhance your website's SEO performance. This guide covers setting up your account and using insights to improve your content strategy.
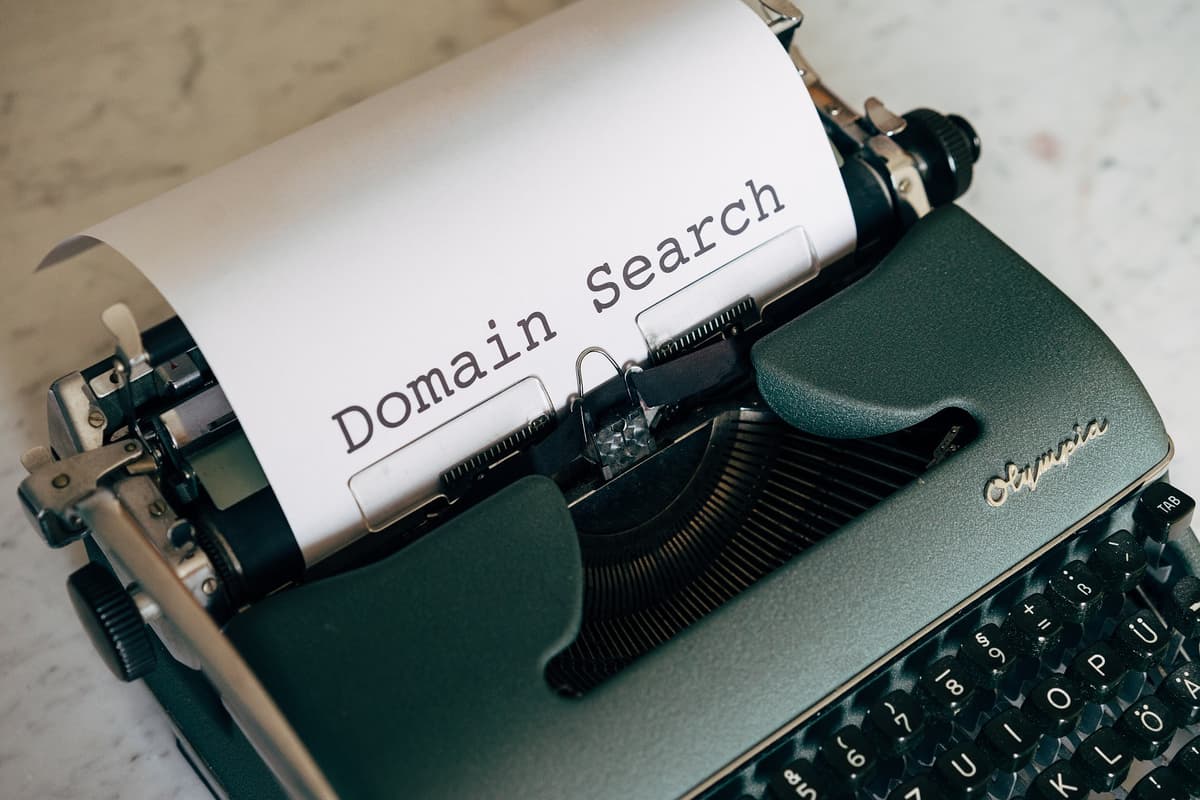
Domain Names: What They Are and How to Choose One
January 12, 2025
Learn about domain names, their structure, and the importance of choosing the right one for your online presence. This post covers best practices for selecting domain names that enhance branding and SEO.
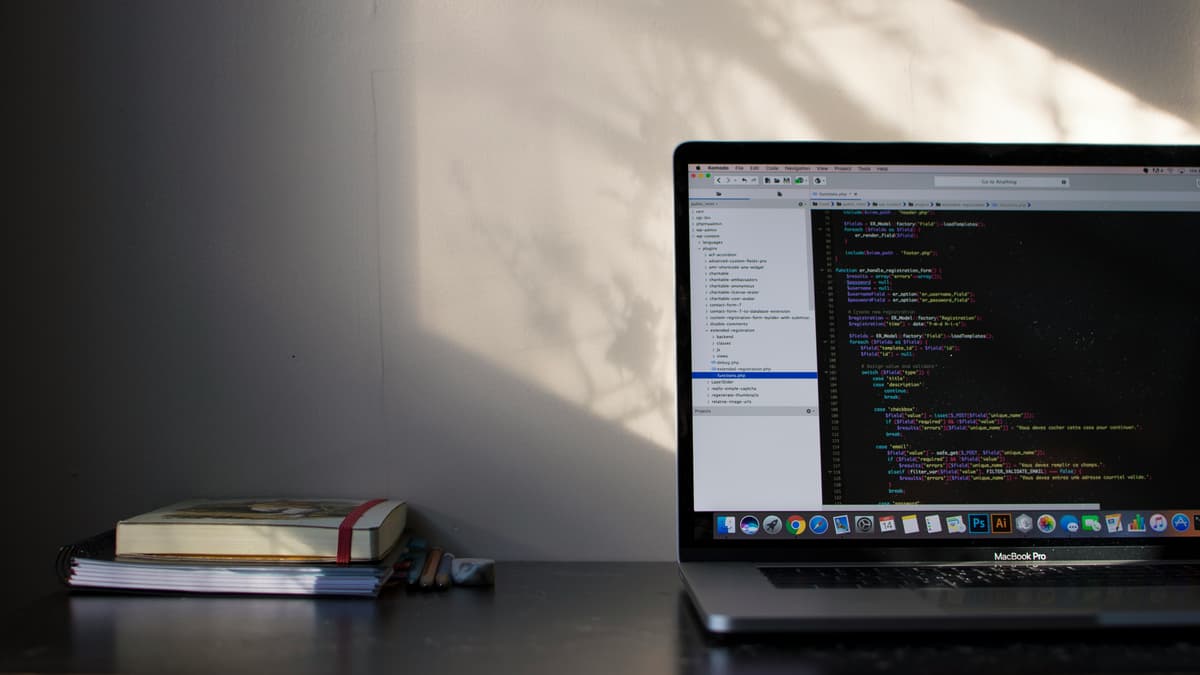
Web Hosting: A Simple Guide to Choosing the Right Provider
January 2, 2025
This guide provides an overview of different types of web hosting services, including shared, VPS, dedicated, cloud, managed, and colocation hosting. It offers practical examples of providers, tips for avoiding scams, and guidance on choosing the right service for your needs. Additionally, it highlights free hosting options like GitHub Pages, Netlify, and Vercel, along with steps for hosting a website that uses HTML, CSS, and JavaScript.
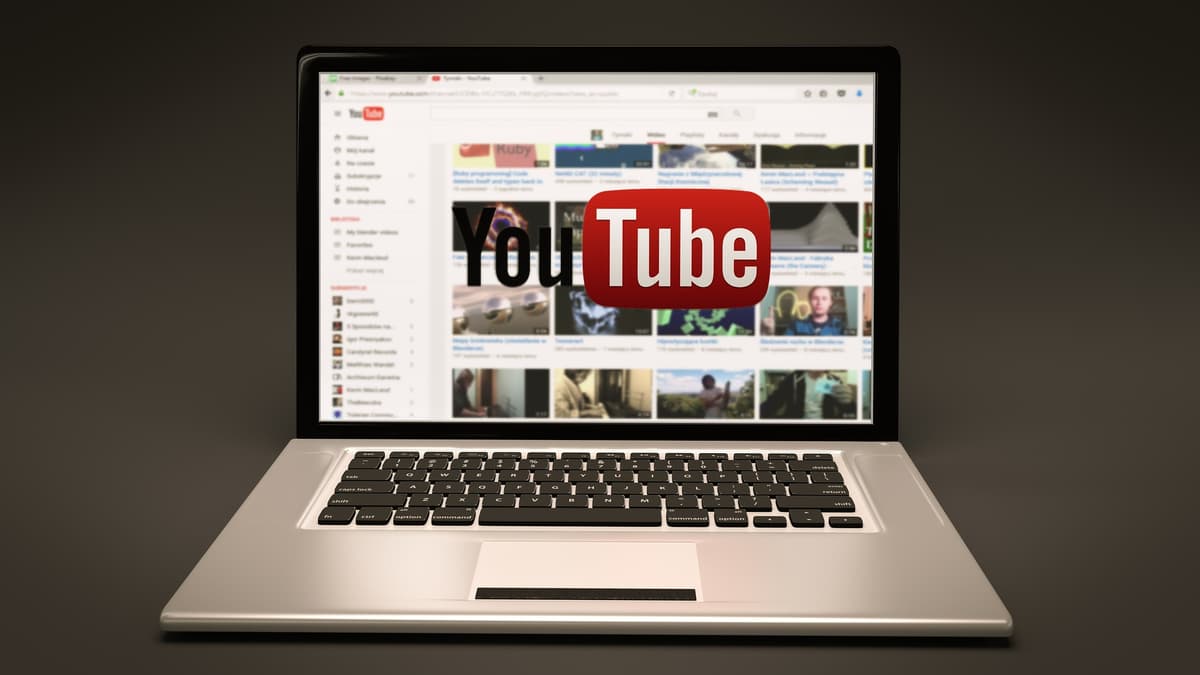
Online Courses and Starting a Tech YouTube Channel: Sharing Your Knowledge and Impacting Lives
January 1, 2025
Learn how to create and sell online courses to share your expertise, and explore the steps to start a tech YouTube channel that can reach and engage a global audience. This blog covers the benefits of online courses, planning content, and strategies to grow a successful YouTube channel.
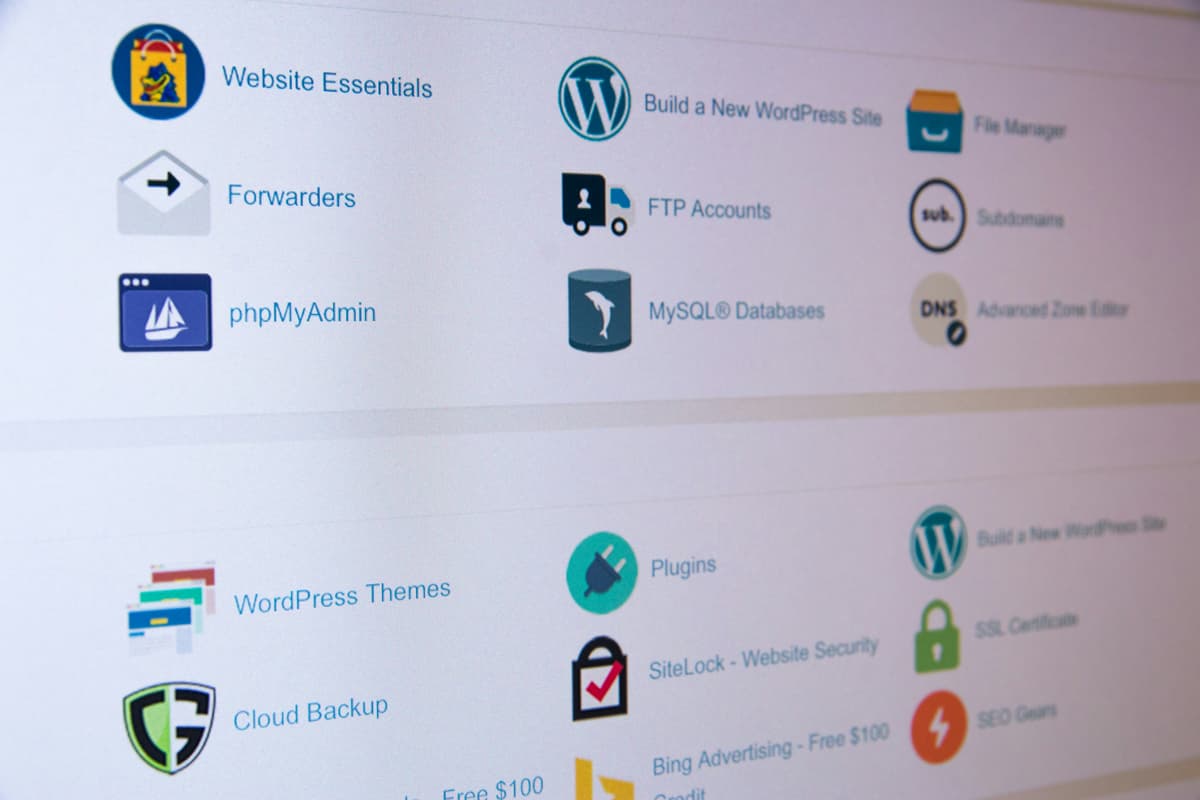
Unleashing the Power of SSL Certificates: Why SSL Matters for Your Website
January 10, 2025
Learn about SSL certificates and their importance in protecting websites. Understand data encryption, authentication, and the types of SSL certificates available, including Domain Validation, Organization Validation, and Extended Validation SSL. Discover how SSL boosts user trust and search engine rankings while ensuring legal compliance.
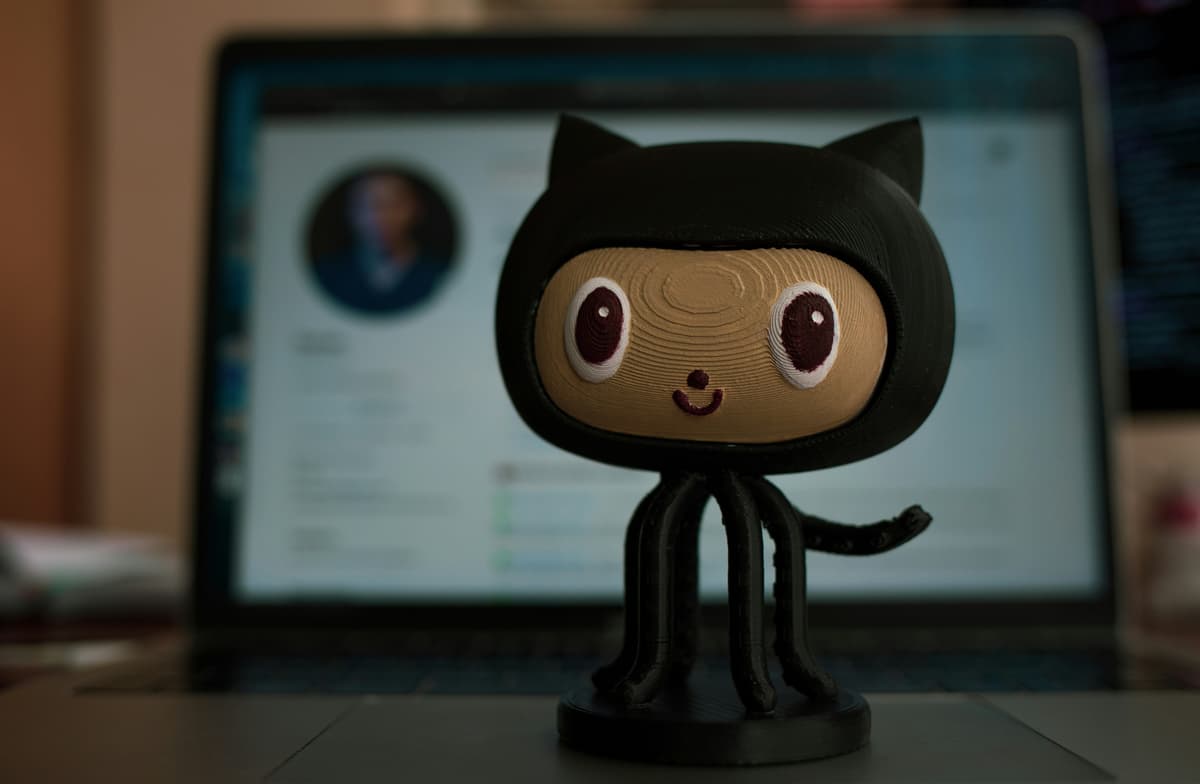
The Importance of Version Control in Software Development
January 9, 2025
Explore the critical role of version control systems like Git in software development. Understand how version control helps manage changes, collaborate with teams, and maintain project history.
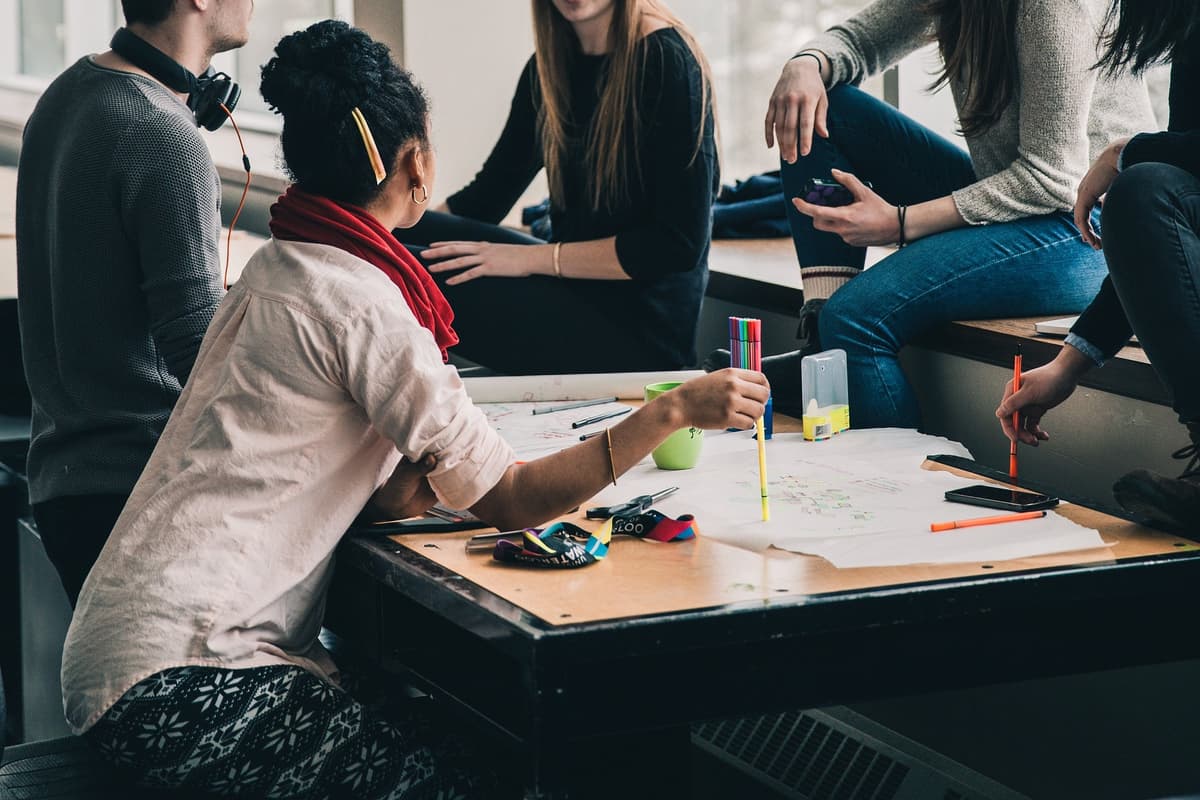
Building Networks as Developers: A Comprehensive Guide to Professional Connections
January 4, 2025
Learn how to build a strong network as a developer and maximize your online presence.
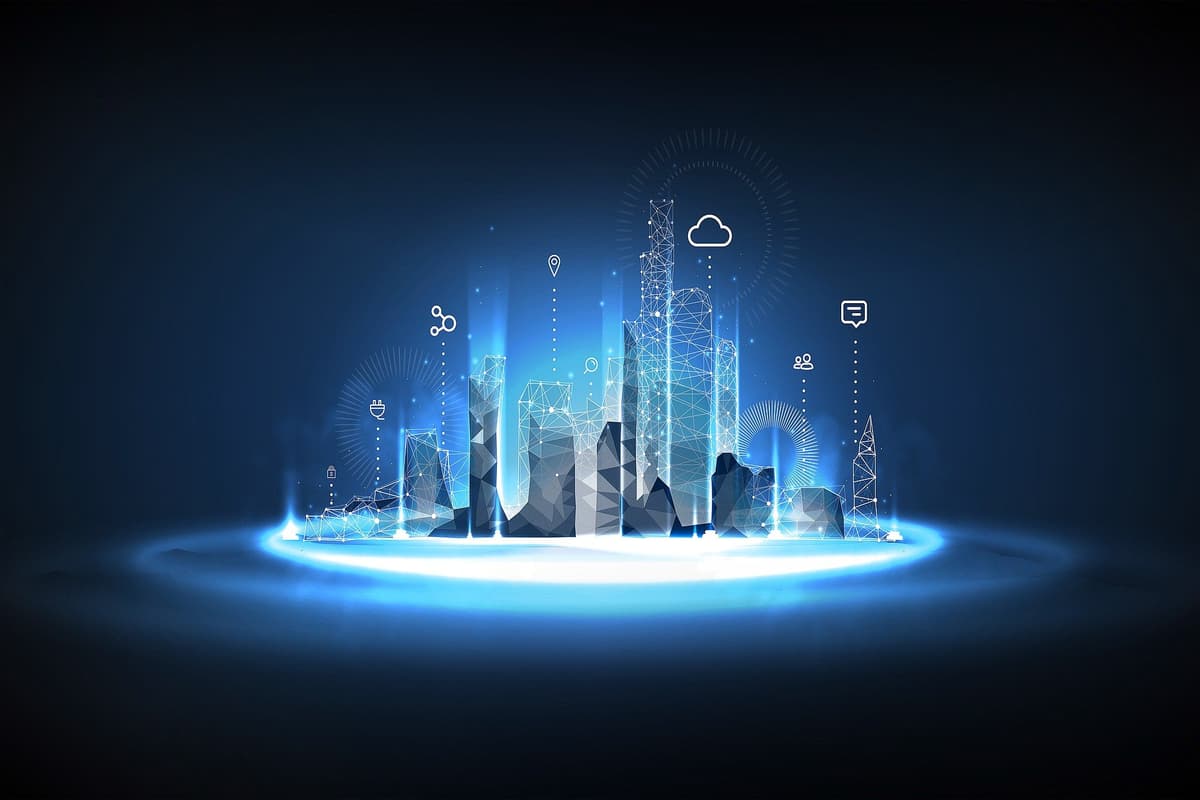
The Internet of Things (IoT): Revolutionizing Our Connected World
January 5, 2025
The Internet of Things (IoT) is transforming the way we live and work by connecting everyday devices to the internet, allowing them to exchange data. This article explores IoT, its history, architecture, real-world applications, and its future impact on industries like healthcare, agriculture, and smart cities. It also discusses the role of AI in IoT, security challenges, and ethical implications.