Cracking the Code: The Essential Framework to Ace Google’s Software Engineering Interviews
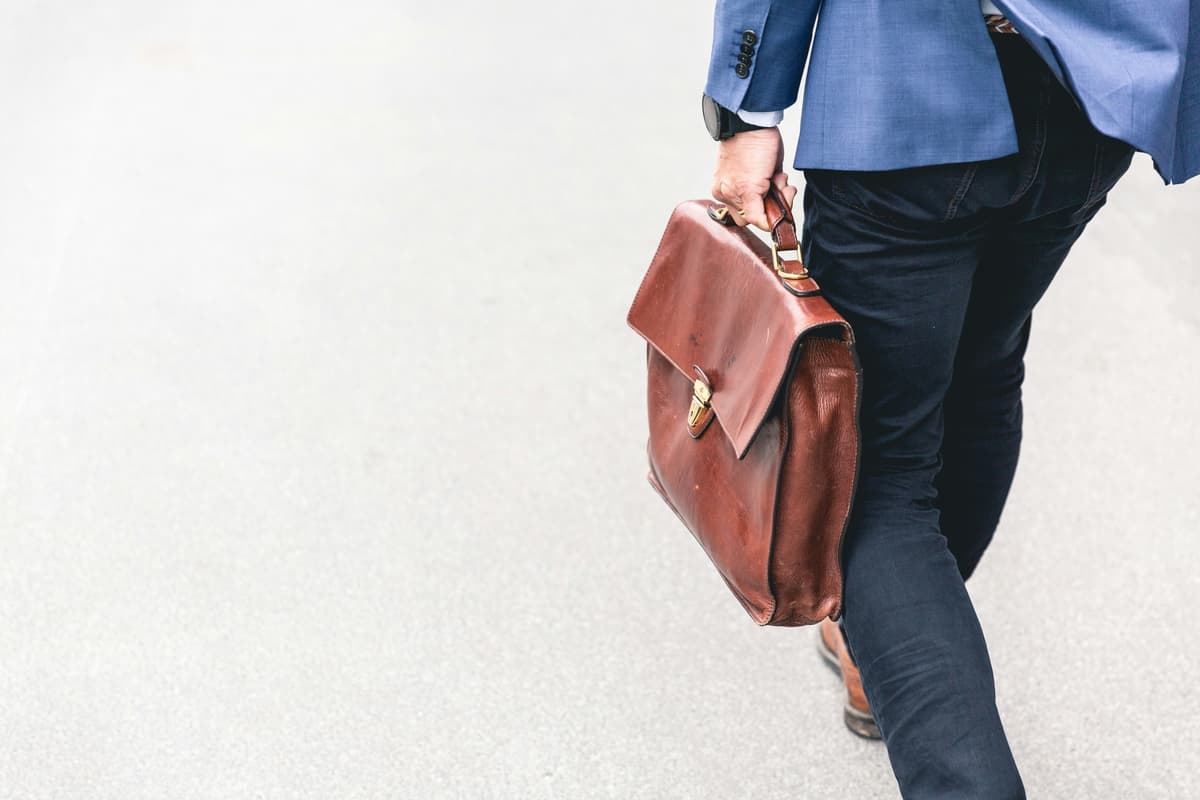
Written by Massa Medi
Landing a job as a software engineer at Google is a feat more difficult than getting into Harvard—less than 1% of all applicants actually receive an offer. Most aspiring engineers believe the key to success is grinding through hundreds of LeetCode problems, but the reality is more nuanced. You’ll find countless stories of people who solved 300, 400, or even 500 coding questions, only to stumble and fall in the real interview.
So, what’s missing from their preparation? Let’s break it down with a familiar scenario: Remember that big exam where you knew the answer to a question, but your mind blanked out until you stepped out of the classroom? That frustrating disconnect is exactly what torpedoes many highly-prepared candidates during technical interviews. Whether you’re a beginner learning code or a seasoned developer, there’s a secret framework you need—a practical approach that will get you unstuck and boost your confidence, no matter what interview hurdle comes your way.
At the heart of this framework are three crucial stages: planning your code, writing your code, and explaining your code. Mastering each stage is vital not just for communicating your solution, but for showing that you can organize thoughts clearly—the skill that separates great candidates from average ones.
Stage 1: Planning Your Code
The first—and perhaps most overlooked—step in nailing a coding interview is effective planning. We can break this down into two main components: clarifying the problem and explaining your algorithm.
Clarifying the Problem
Imagine yourself nervously signing on to a Zoom interview. The interviewer joins and outlines the coding problem you need to tackle. It may be tempting to jump straight into writing code or to make a quick guess at the solution. Don’t! If you misunderstand the problem or work on the wrong task, you’re setting yourself up for an immediate rejection.
Instead, take a breath and clarify the problem. This starts with providing your own sample inputs and outputs. For example, if you're asked to reverse a string, state a test case out loud: “If the input is ‘abc’, the output should be ‘cba’—is that correct?” This confirms your understanding and demonstrates strong communication skills.
Next, probe further by asking about at least three edge cases. Consider things like the size of the input, how to handle negative numbers, or empty arrays. This not only shows you’re a thorough problem-solver, it also helps you spot any tricky scenarios before you get blindsided. Once you’ve nailed down the problem definition, you’re ready to discuss how to solve it.
Explaining Your Algorithm
Here’s a common pitfall: leaping directly to the most optimal, elegant solution. After months of LeetCode prep, it’s easy to get caught in the trap of perfection. But, ironically, this can work against you. The real trick is to start simple.
Propose a straightforward, even naive approach first. For instance, if the problem could be solved with either a linear or binary search, start by discussing the linear approach—“Should I try linear search first to validate the problem before optimizing?” You might be surprised: sometimes, the interviewer is only looking for a working solution!
If they signal that a more advanced answer is needed, only then proceed to optimize. And if you’re struggling to instantly recall the best algorithm, honestly assess whether more practice is what you need, or if it’s just nerves talking. For those still building the basics, the widely recommended “LeetCode 75” list is the perfect starting point.
Above all, when formulating your solution, say you’re dropping restrictions or tackling a simpler case first—make this explicit. Walk the interviewer through test cases and potential algorithms. This not only clarifies your thought process but also invites the interviewer to collaborate and offer guidance.
Once you’ve identified your approach, write down your algorithm in comments or as bullet points in your editor. Outline the data structures you plan to use and which algorithms will get you from input to answer. Narrate your thought process as you do this and check with your interviewer for questions or feedback. If they’re on board, you’re cleared to begin coding!
Stage 2: Writing Your Code
Now, as you jump into writing code, project confidence. In this phase, interviewers generally won’t interject—they want to see how you translate ideas into working code, and how closely you stick to your outline. Their focus? Your communication and coding efficiency.
Here’s a real anecdote to drive the point home: I once interviewed a master’s student with several impressive internships behind him. He nailed the outline, identified the optimal algorithm, and clearly articulated his plan. Then, as soon as he started typing, he went silent—totally focused on the code. Even though he got stuck parsing a string, he persisted and finished in time. I gave him a pass for his problem-solving, but the other interviewers didn’t. Their reason? He didn’t communicate enough after the planning stage.
Tips for Communicating While Coding
- Talk as you code: Even a running commentary of your actions helps (“Now I’m sorting the array to find the largest element. I’ll use a standard library sort. Once sorted, I’ll return the last element.”).
- If multitasking is tough, alternate: Briefly explain what you plan to code next, tie it to your earlier outline, then code it silently.
- Highlight code choices: Reference variable names, data structures, or notable logic to keep the interviewer engaged in your process.
Efficiency—how quickly and clearly you write code—matters too. Choose good variable names and aim for readable, logically organized code. But here’s a universal truth: everyone gets stuck somewhere. Whether it’s a bizarre error, a tricky function, or an unexpected edge case, how you react could make or break your interview.
How to Handle Getting Stuck
- Don’t waste precious time: If stumped, leave a brief
// TODO
in your code and move on to the next part of your algorithm. You can always return to these mini roadblocks if time permits. - For minor checks (like null validation): Mark them as
// TODO: null check
. More often than not, interviewers don’t require exhaustive edge handling in a 30–45 minute window—but if they do, address them at the end.
Stage 3: Explaining Your Code — Prove It, Optimize It, or Both
As you finish, realize the interviewer is now assessing your “level”—are you a junior who’s just scrambling to get code working, or a future senior who can explain, optimize, and teach? The difference? Proactive, clear explanation.
“If you can’t explain it simply, you don’t understand it well enough.” — Albert Einstein
When your code is written, you face a fork in the road: Should you test your solution or work on optimizing it further? Here’s a simple fix—just ask your interviewer what they want to see next.
- If asked to optimize: Revisit your code. Identify and circle (figuratively or with notes) the bottleneck—the section where your runtime is highest. Communicate each part’s Big O complexity, then propose and explain improvements. This shows advanced reasoning and technical mastery.
- If your solution is accepted: Prove your code! Pick a realistic test case (ideally one of the simple inputs or edge cases discussed earlier) and manually walk through your code, line by line, demonstrating how the input is transformed into the correct output.
A key interview pitfall: Don’t use overly large or complex test cases for your demo. You simply won’t have time to walk through every detail, and you risk confusing both yourself and your interviewer. Stick to small, digestible scenarios that clearly show your algorithm in action.
Final Thoughts: Your Roadmap to a Google Offer
If you methodically apply this three-stage framework—planning with clarity, coding with transparency, and explaining with confidence—you dramatically increase your chance of joining that elusive 1% who get the Google offer. It’s not just about brute-forcing practice problems. It’s about demonstrating mastery of your own thought process, flawless communication, and the ability to solve problems collaboratively.
Remember: Before you type a single line of code, show you understand the problem. Walk the interviewer through your thinking, invite clarifications, and never be afraid to start simple before refining your solution. Communicate every step—not just in your code but in your voice. And when you’re finished, prove your answer with a clear, simple dry run.
In the end, that’s what turns interviewers’ heads and earns you a seat at Google. Happy coding—and may your next interview be your last!